Bildirim
AVR-GCC DE YAZILAN PROGRAMLAR
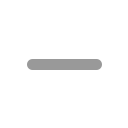

Daha Fazla 
Bu Konudaki Kullanıcılar:
Daha Az 

2 Misafir (1 Mobil) - 1 Masaüstü,
1 Mobil


Giriş
Mesaj
-
-
#include <avr/io.h>
#ifndef __LCD_H
#define __LCD_H
#define NOP() asm volatile ("nop" ::)
#define LCD_RS_PIN 0 //LCD Register Select Pin
#define LCD_RS_PORT PORTD
#define LCD_RS_DDR DDRD
#define LCD_CS_PIN 1 //LCD Chip Select Pin
#define LCD_CS_PORT PORTD
#define LCD_CS_DDR DDRD
#define LCD_DATA_PORT PORTB //LCD Data Port
#define LCD_DATA_DDR DDRB
#define LCD_DATA_PIN PINB
#define LCDCS_ON() { sbi(LCD_CS_PORT,LCD_CS_PIN); }
#define LCDCS_OFF() { cbi(LCD_CS_PORT,LCD_CS_PIN); }
#define LCDRS_ON() { sbi(LCD_RS_PORT,LCD_RS_PIN); }
#define LCDRS_OFF() { cbi(LCD_RS_PORT,LCD_RS_PIN); }
extern void LCDInit(void);
extern void LCDWriteByte(unsigned char);
extern void LCDWrite(char *);
extern void LCDCls(void);
extern void LCDPos(unsigned char, unsigned char);
extern void ShowHex(unsigned char by);
extern void ShowHexLong(unsigned long l);
extern void Delay1ms(unsigned int);
extern void Delay100us(unsigned char);
#endif
#define sbi(portn, bitn) ((portn)|=(1<<(bitn)))
#define cbi(portn, bitn) ((portn)&=~(1<<(bitn)))
//*******************************************
// Schreibt ein Datenbyte in die LCD-Anzeige
//*******************************************
void LCDWriteByte(unsigned char data)
{
LCDCS_OFF();
//4 Bit Operation
LCD_DATA_PORT &= 0x0F; //LCD Data Port D7..3 zero
LCD_DATA_PORT |= (data & 0xF0); //High Nibble des Bytes
LCDCS_ON();
LCDCS_OFF();
LCD_DATA_PORT &= 0x0F; //LCD Data Port D7..3 zero
LCD_DATA_PORT |= ((data << 4) & 0xF0); //Low Nibble des Bytes
LCDCS_ON();
LCDCS_OFF();
Delay100us(0); //etwas auf die Anzeige warten
}
//**************************************************
//Schreibt einen Text in die LCD-Anzeige
//**************************************************
void LCDWrite(char *__putstr)
{
char __ps;
while((__ps = *__putstr))
{
__putstr++;
if (__ps== 0) break;
LCDWriteByte(__ps);
}
}
//****************************************************
// Springt auf Zeile x und Position y der LCD-Anzeige
// Gültig für 2 Zeilen
//****************************************************
void LCDPos(unsigned char zeile,unsigned char position)
{
unsigned char buff;
LCDRS_OFF(); //Umschalten auf Kontrollregister
// 0x80 wegen Display Adress Set.
// Erste Position ist bei mir 1, nicht 0.
// Offset's
// Zeile 1 -> 0x00+0x80-0x01=0x7F
// Zeile 2 -> 0x40+0x80-0x01=0xBF
switch(zeile)
{
case 1: buff=0x7F+position; break;
case 2: buff=0xBF+position; break;
default : buff=0x80; break; //1,1
}
LCDWriteByte(buff);
LCDRS_ON(); //Umschalten auf Datenregister
Delay100us(1); //etwas auf die Anzeige warten
}
//*****************************************
// Löscht die LCD Anzeige
// Cursor steht dann auf Position 1,Zeile 1
// Gilt auch für mehrzeilige Anzeigen
//*****************************************
void LCDCls(void)
{
LCDRS_OFF(); //Controlregister
LCDWriteByte(0x01); //CLS
LCDRS_ON(); //Umschalten auf Datenregister
Delay1ms(1);
}
//*********************************************
//Stellt die Funktionsweise der LCD Anzeige ein
//*********************************************
void LCDInit(void)
{
int i;
sbi(LCD_RS_DDR,LCD_RS_PIN); //LCDRS is output
sbi(LCD_CS_DDR,LCD_CS_PIN); //LCDCS is output
//4 Bit Operation
LCD_DATA_DDR|=0xF0; //All outputs D7..3
LCD_DATA_PORT&=0x0F; //LCD Data Port D7..3 zero
LCDRS_OFF(); //Control Register
LCDCS_OFF(); //LCD-Anzeige anwählen
//Software Reset laut Hitachi Datenblatt
Delay1ms(1); //Möglichen Power On Reset abwarten
for(i=0; i<3; i++)
{
//4 Bit Operation
LCD_DATA_PORT&=0x0F; // LCD Data Port D7..3 zero
LCD_DATA_PORT|=0x30;
LCDCS_ON(); //schreiben
LCDCS_OFF(); //LCD-Anzeige abwählen
Delay1ms(1); //Ein bißchen warten auf die Anzeige
}
//Ende Software Reset
//4 Bit Operation
//Der erste Schreibzugriff nach dem Reset ist ein 8 Bit Zugriff!!
//Also nur einmal schreiben, nicht Low-High Nibble.
//4Bit Operation einschalten
LCD_DATA_PORT &= 0x0F; // LCD Data Port D7..3 zero
LCD_DATA_PORT |= 0x20;
LCDCS_ON(); //High Nibble schreiben
LCDCS_OFF();
Delay1ms(1);
LCDWriteByte(0x28); //4 Bit Operation,2 Line,5x7 Font
Delay1ms(1);
LCDWriteByte(0x14); //Cursor Move,Right Shift
Delay1ms(1);
// LCDWriteByte(0x0F); //Display on, Cursor on ,Cursor blink
// LCDWriteByte(0x0E); //Display on, Cursor on ,Cursor no blink
LCDWriteByte(0x0C); //Display on, Cursor off ,Cursor no blink
Delay1ms(1);
LCDWriteByte(0x06); //Increment, Display Freeze
Delay1ms(1);
LCDWriteByte(0x02); //Cursor Home
Delay1ms(1);
LCDRS_ON(); //LCD-Datenregister
}
//#######################################################
//Zeigt ein Byte im HexCode (ohne 0x vorne) an
void ShowHex(unsigned char by)
//#######################################################
{
unsigned char buff;
buff=by>>4; //Highnibble zuerst
if(buff<10) buff+='0'; //ASCII Code erzeugen
else buff+=0x37; //Großbuchstaben
LCDWriteByte(buff);
buff=by&0x0f; //Danach das Lownibble
if(buff<10) buff+='0'; //ASCII Code erzeugen
else buff+=0x37; //Großbuchstaben
LCDWriteByte(buff);
}
//#######################################################
void ShowHexLong(unsigned long l)
//#######################################################
{
ShowHex((unsigned char)(l>>24));
ShowHex((unsigned char)(l>>16));
ShowHex((unsigned char)(l>>8));
ShowHex((unsigned char)(l));
}
//###################################################################################
//Nicht sonderlich genau !!
void Delay1ms(unsigned int time)
//###################################################################################
{
unsigned int i;
for(i=0; i<time; i++) Delay100us(10);
}
//###################################################################################
//Nicht sonderlich genau !!
//time<=255 !!
void Delay100us(unsigned char time)
//###################################################################################
{
unsigned char k,l;
for(k=0; k<time; k++)
{
for(l=0; l<40; l++)
{
//Ein CPU-Clock = 0.125us bei 8MHz
NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP();
NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP();
#ifdef F_11059
NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP();
#endif
#ifdef F_16
NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP();
NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP(); NOP();
#endif
}
}
}
int main( void )
{
// Setup PortA
PORTA = 0x00;
DDRA = 0x00;
// Setup PortB
PORTB = 0x01;
DDRB = 0x87;
// Setup PortC
PORTC = 0x00;
DDRC = 0x00;
// Setup PortD
PORTD = 0x06;
DDRD = 0x00;
LCDInit();
LCDCls();
LCDWrite("Hello");
LCDPos(2,1);
LCDWrite("world");
for(;;)
{
if (!( PINC & (1<<PINC0) )) LCDWrite("rezistor");
if (!( PINC & (1<<PINC1) )) LCDWrite("REZISTOR");
}
}
programı denedim çalışıyor fakat LCDWrite() fonksiyonuna integer tipte değişken gönderilmiyor
önce integer dan string e çevirmek gerekiyor böyle bir fonksiyon bilen arkadaş varsa foruma eklerse sevinirim...
teşekkürler...
-
arkadaşlar bu sorunuda çözdüm programın başına ( extern char *itoa(int __val, char *__s, int __radix); )
bu satırı yazın fonksiyon gibi kullanın örneğin ;
int A;
char B[10];
A=123;
itao(A,B);
diyerek char tipini LCDWrite(); fonksiyonuna gönderin. böylece adc çevirim sonucu vs... lcd de görebilirsiniz...
başka program yazan arkadaşlar varsa eklesinler lütfen...
bu arada osc=8MHz dir....
Sayfa:
1
Ip işlemleri
Bu mesaj IP'si ile atılan mesajları ara Bu kullanıcının son IP'si ile atılan mesajları ara Bu mesaj IP'si ile kullanıcı ara Bu kullanıcının son IP'si ile kullanıcı ara
KAPAT X
Bu mesaj IP'si ile atılan mesajları ara Bu kullanıcının son IP'si ile atılan mesajları ara Bu mesaj IP'si ile kullanıcı ara Bu kullanıcının son IP'si ile kullanıcı ara
KAPAT X